오류를 다루는 방법들. Exception, Logging, Debug.Assert
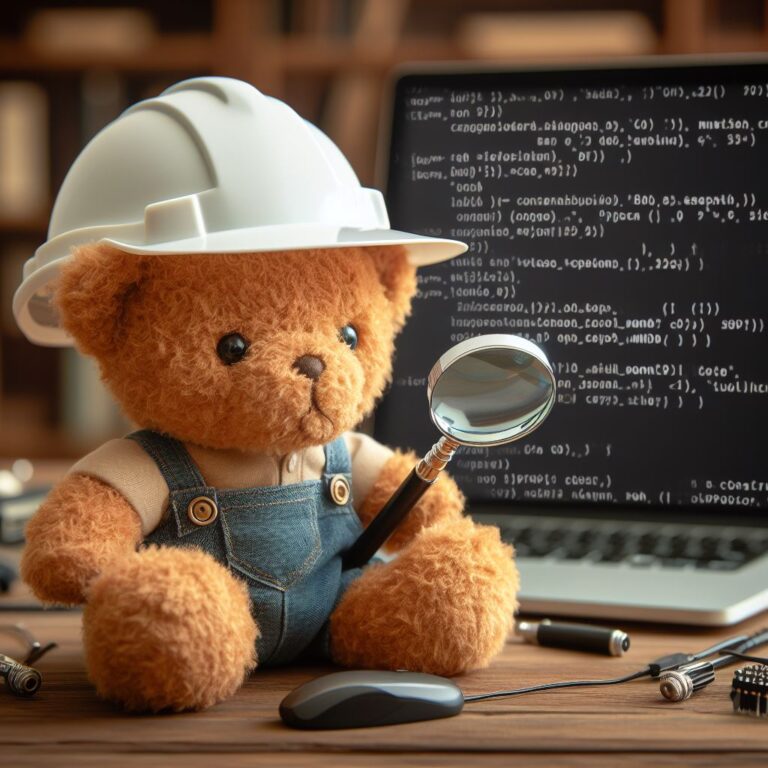
Debug.Assert, 예외 처리(exception handling), 그리고 로깅(logging)은 모두 프로그램의 오류를 다루는 방법이지만, 사용 목적과 적용 시점이 다릅니다. Debug.Assert Debug.Assert는 개발자가 코드의 불변성(invariants)을 가정하는 부분에서 사용합니다. 주로 디버그 모드에서만 활성화되며, 릴리스 모드에서는 제거되어 성능에 영향을 주지 않습니다. Debug.Assert는 “이것은 절대 발생하지 않아야…